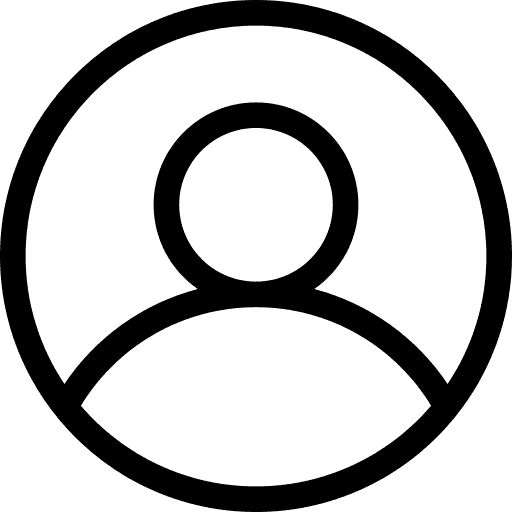
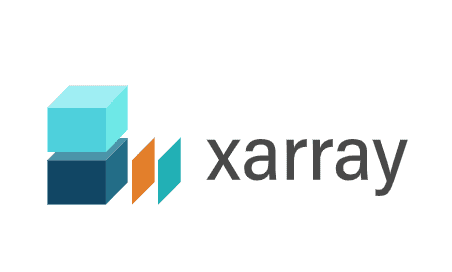
Abstract
Managing multi-dimensional datasets can be complex, especially with traditional libraries like NumPy and Pandas. Xarray is a powerful Python library that addresses these challenges. It extends NumPy by enabling multi-dimensional arrays with labeled dimensions and coordinates, making data more readable and easier to manipulate. This blog explores the problem of handling multi-dimensional data, how Xarray provides a robust solution, and offers a practical implementation guide.
Solution Details
Xarray addresses these issues by providing labeled multi-dimensional arrays, making data management and analysis both efficient and intuitive.
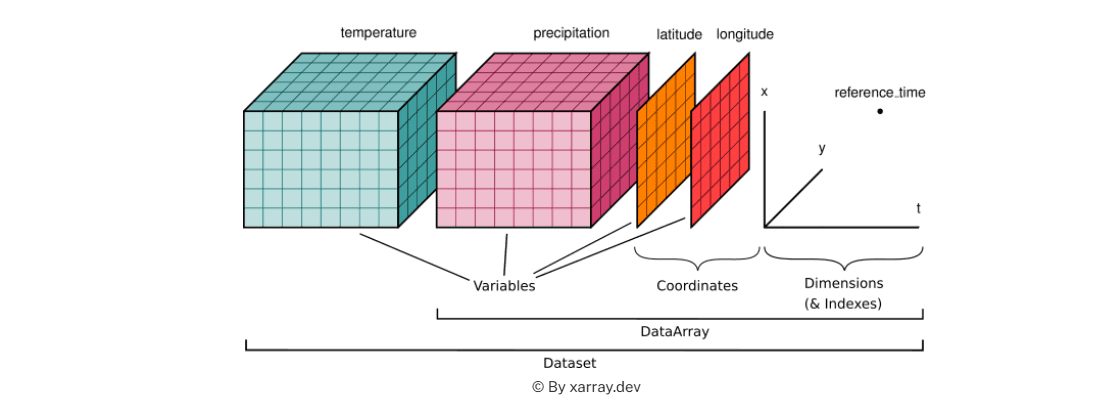
Xarray which is built upon pandas and NumPy provides two main data structures.
- DataArrays that wrap underlying data containers (e.g. NumPy arrays) and contain associated metadata
- DataSets that are dictionary-like containers of DataArrays. It is very similar to the pandas’ data frame.
Code/Implementation Steps
For a practical example, let’s go through reading a netCDF file and performing some simple analysis using Xarray.
-
Importing a NetCDF file
To import data from a NetCDF file, use the open_dataset() method. You can also import multiple files at once in a single dataset using the open_mfdataset().
import xarray as xr try: with xr.open_dataset('./temperature.nc') as ds: print(ds) except Exception as err: print('oops...', err)
- import xarray as xr imports the Xarray library, which is used for handling multi-dimensional arrays in a user-friendly way.
- The xr.open_dataset() function in Xarray is used to open and load datasets from various file formats, such as NetCDF, HDF5, GRIB, and more.
-
Extract and Query Data
You can extract data from a particular variable simply using the dot operator. ds.data_array_name
ds.lat
You can also query the dataset, using where()
ds.where(ds.temperature < -1)
- This provides a quick way to extract specific variables and filter data based on conditions in an Xarray dataset.
-
Convert any Xarray dataset to a Pandas DataFrame
To convert any Xarray dataset to a Pandas DataFrame, you can use to_dataframe() method
ds.to_dataframe()
- Once you have a DataFrame you can apply any methods from pandas on it to get different views on the data.
-
Dealing with Multiple datasets
Here’s how you can open multiple datasets at once and convert them to a DataFrame
files_to_collate = ['temperature.nc', 'humidity.nc'] filters = 'temperature <= 0 & humidity > 50' with xr.open_mfdataset(files_to_collate) as ds: df = ds.to_dataframe().dropna(how="all") filtered_df = df[df.eval(filters)] print(filtered_df)
- The eval() function evaluates a string describing operations on Pandas DataFrame columns.
- The resulting DataFrame has columns from both the dataset variables, mapped against the coordinates variables
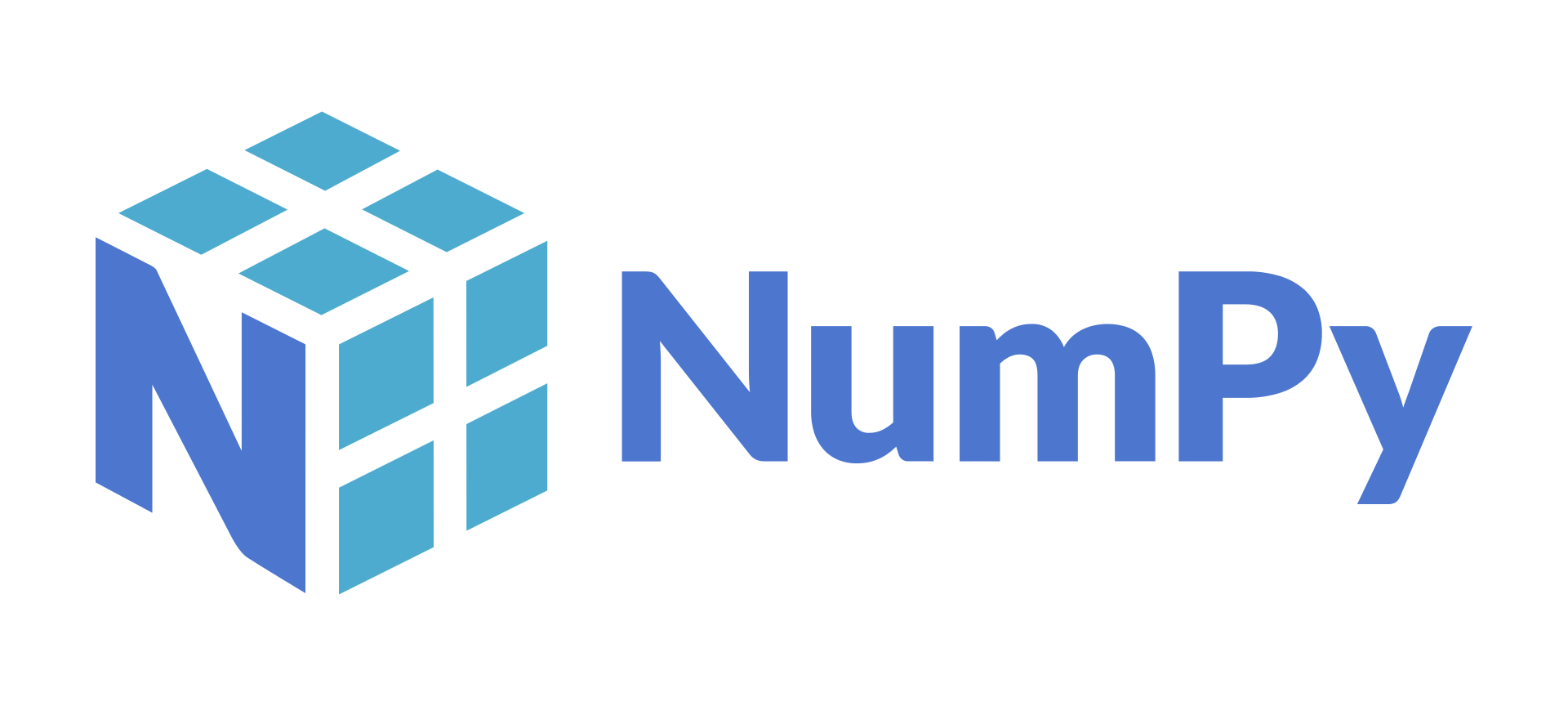
Conclusion
Xarray is an incredibly powerful tool for working with multi-dimensional data. By providing labeled arrays and datasets, it simplifies the process of data analysis, making it easier to manipulate, slice, and visualize data.