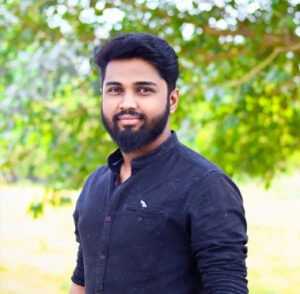
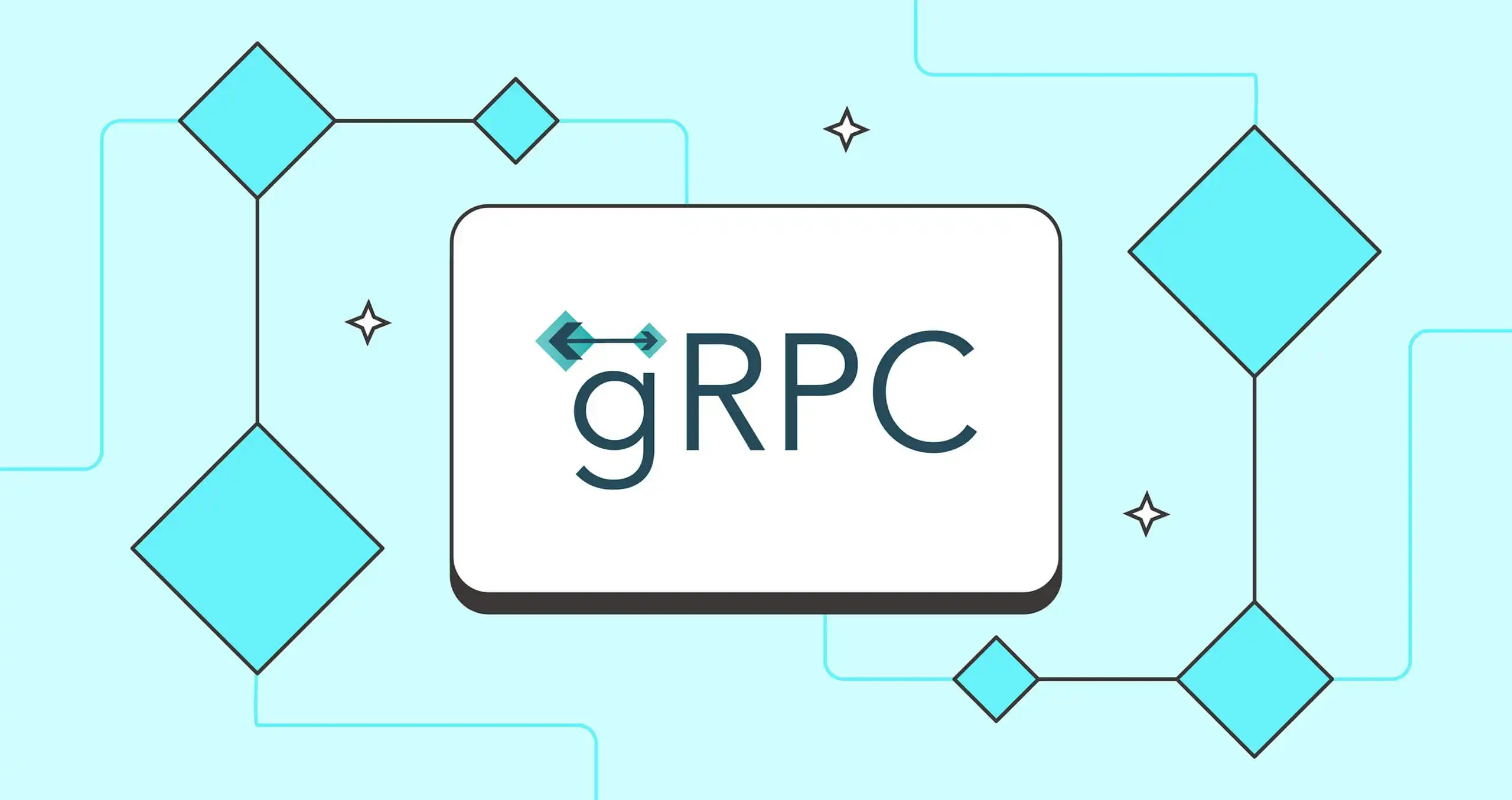
Abstract
gRPC (gRPC Remote Procedure Call) is a high-performance, open-source remote procedure call (RPC) framework developed by Google basically to connect with a large number of microservices. It uses Protocol Buffers, a binary serialization format, to define and serialize APIs and messages sent between client and server applications.
Developers can define services using Protocol Buffers, and gRPC generates server and client code in multiple languages, making it easier to create distributed applications that can communicate with each other seamlessly. One key feature is its support for bi-directional streaming, which can result in significant performance improvements
Solution Details
This solution demonstrates how to set up a basic gRPC communication system using Node.js. It involves creating both a server and a client based on Protocol Buffers to facilitate efficient and strongly-typed data exchange.
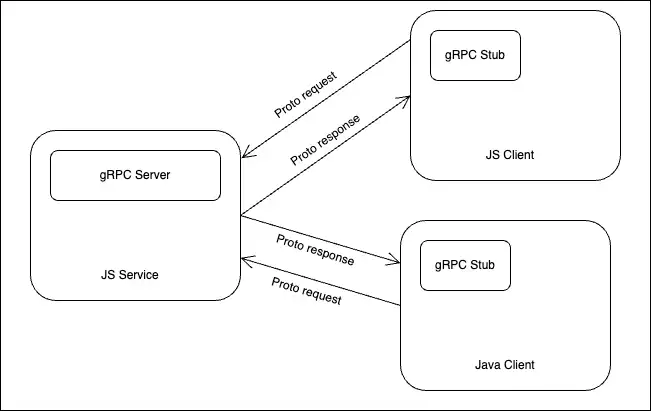
HTTP/2
HTTP/2 (Hypertext Transfer Protocol version 2) is a major revision of the HTTP network protocol used to transfer data between a web server and a web browser. It was developed by the HTTP Working Group of the Internet Engineering Task Force (IETF) and was published in May 2015.
HTTP/2 was designed to address the limitations and performance issues of HTTP/1.1, which has been the predominant protocol used on the web since 1999.
Key features
- Multiplexing: HTTP/2 allows for multiple requests and responses to be sent and received simultaneously over a single TCP connection. Which helps to reduce latency.
- Server Push: HTTP/2 enables the server to push resources to the client before they are requested, reducing the need for the client to make additional requests.
- Binary protocol: HTTP/2 uses a binary protocol instead of the text-based protocol used by HTTP/1.1, which simplifies parsing and reduces overhead
- Header Compression: HTTP/2 uses header compression to reduce the size of headers, which can significantly reduce the amount of data that needs to be transferred
- Security: HTTP/2 requires the use of Transport Layer Security (TLS), providing improved security and privacy over HTTP/1.1
Versioning
Protocol Buffers (proto3) allows versioning through its schema. You can easily evolve your service over time by adding new fields or services while maintaining backward compatibility. Changes like adding fields are handled gracefully without breaking existing clients.
- gRPC allows versioning of services using Protocol Buffers. As new features are added, backward compatibility is preserved by ensuring old clients still work with the updated server, provided that fields are added, not removed, or renamed.
- To support multiple versions of a service, you can define separate services or methods in different .proto files and migrate gradually.
Integration
The server and client interact using the test.proto service definition, which ensures that both the client and server share a consistent API. Integration with other services, languages, or platforms is simplified, as long as they adhere to the same protobuf schema.
- gRPC provides seamless integration between services, even if they are implemented in different programming languages.
- Both the client and server use the same .proto definition, ensuring they share the same API contract. This is key for integrating microservices or heterogeneous systems.
- gRPC’s language-agnostic nature allows integration between systems written in different languages (e.g., Python, Go, Java).
Code/Implementation Steps
Here’s a sample of Protocol Buffers code for efficient data serialization and communication.
syntax = “proto3”;
package test;
message Request {}
message Response {}
service TestService {
rpc Test (Request) returns (Response);
}
- This Protocol Buffers code defines a simple service with a Test RPC method. The Test method takes a Request message as input and returns a Response message.
-
Setting up the server
Setting up the server involves implementing the service logic and binding it to a network port for handling incoming requests.
const grpc = require("@grpc / grpc - js"); const protoLoader = require("@grpc / proto - loader"); const path = require("path"); const PROTO_PATH = path.join(__dirname, "definitions / test.proto"); const protoDefinition = protoLoader.loadSync(PROTO_PATH, { keepCase: true, longs: String, enums: String, defaults: true, }); const protoDescriptor = grpc.loadPackageDefinition(protoDefinition); const Server = new grpc.Server(); Server.addService(protoDescriptor.test.TestService.service, { test: (call, callback) => { callback(null, "Test"); }, }); Server.bindAsync("0.0.0.0: 50051", grpc.ServerCredentials.createInsecure(), () => { Server.start(); console.log("Server started on port ", 50051); });
- This code sets up a gRPC server using grpc and proto-loader, loading the test.proto file to define the service and methods.
- It binds the server to port 50051 and implements the test method to send a simple "Test" response upon receiving a request.
-
Setting up with client
Setting up the client involves creating a stub to call the remote service method and sending the request to the server.
const grpc = require("@grpc/grpc-js"); const protoLoader = require("@grpc/proto-loader"); const path = require("path"); const PROTO_PATH = path.join(__dirname, "definitions/test.proto"); const protoDefinition = protoLoader.loadSync(PROTO_PATH, { keepCase: true, longs: String, enums: String, defaults: true, }); const protoDescriptor = grpc.loadPackageDefinition(protoDefinition); // Create client with Test Service defined in protobuf const client = new protoDescriptor.test.TestService( "localhost:50051", grpc.credentials.createInsecure(), ); // Call gRPC method client.test(null, () => { console.log("Server responded"); });
- This code creates a gRPC client by loading the test.proto service definition and connecting to a server running on localhost:50051.
- The client calls the test method of the TestService, and logs "Server responded" when the server returns a response.
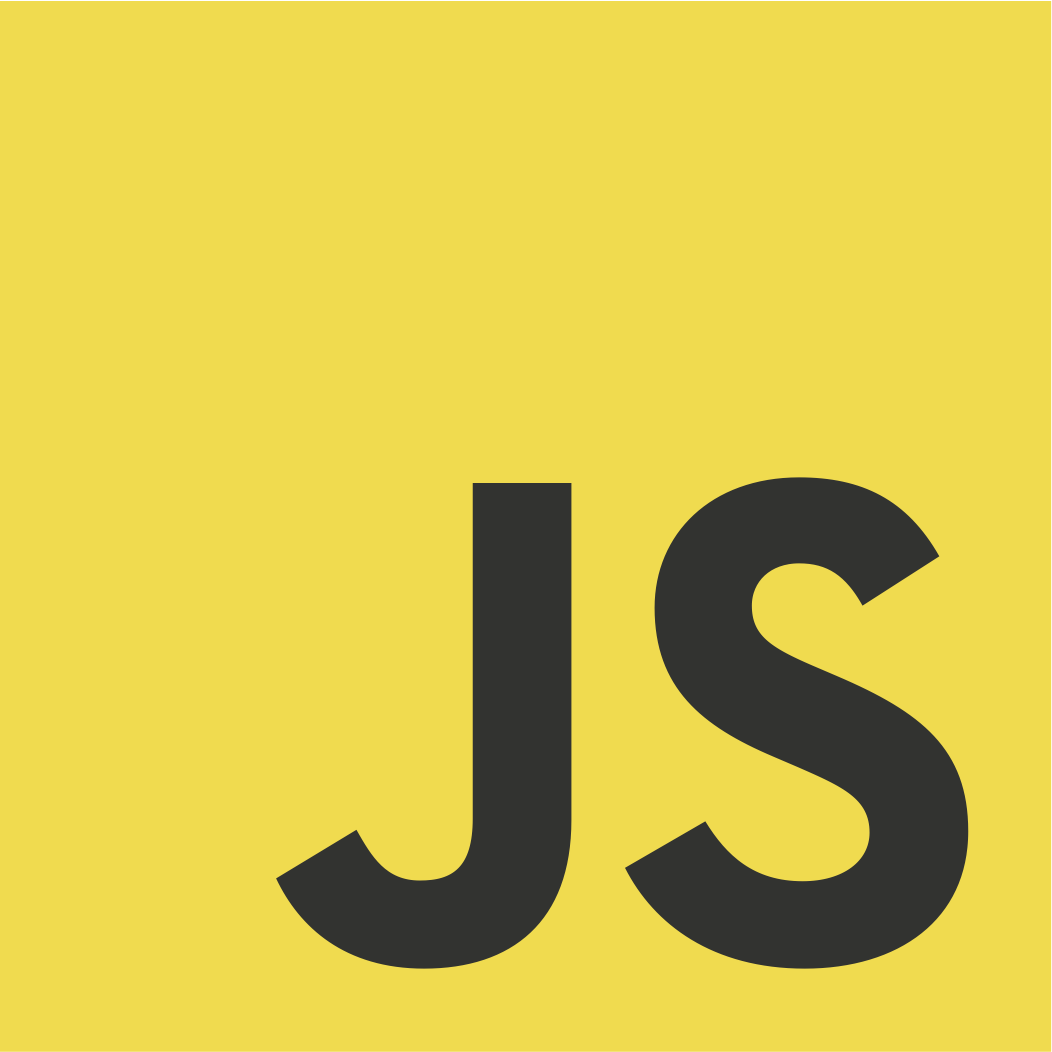
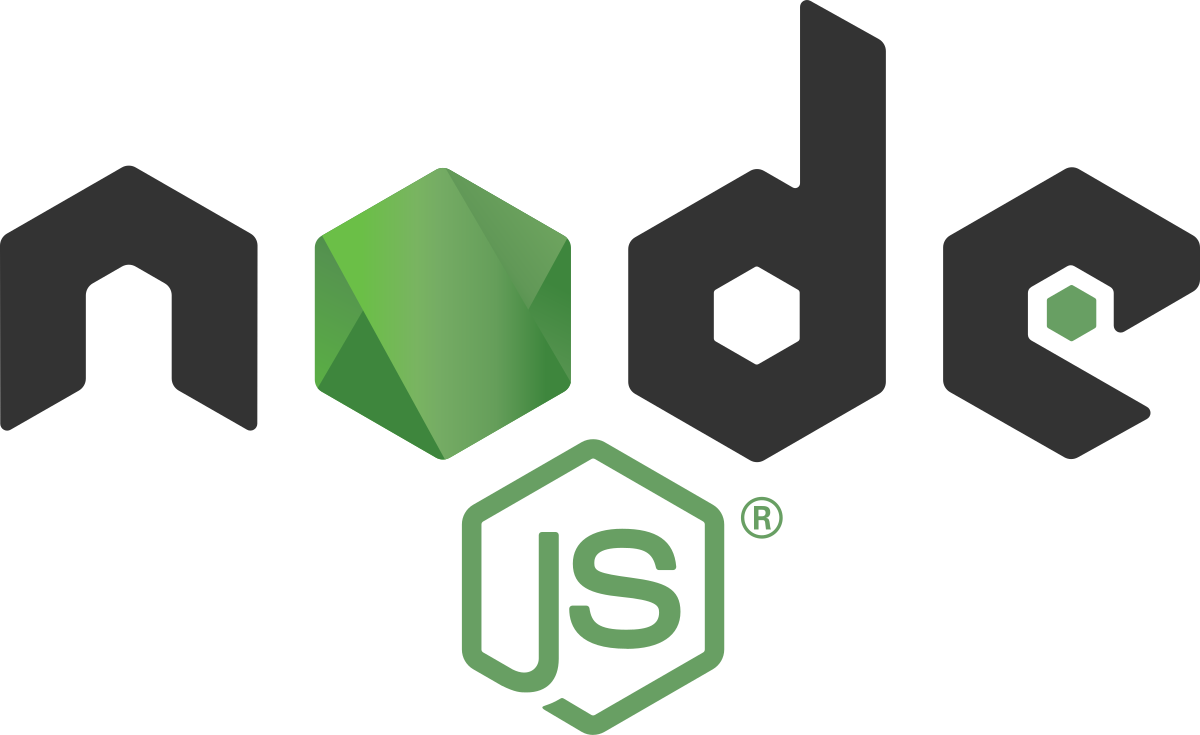
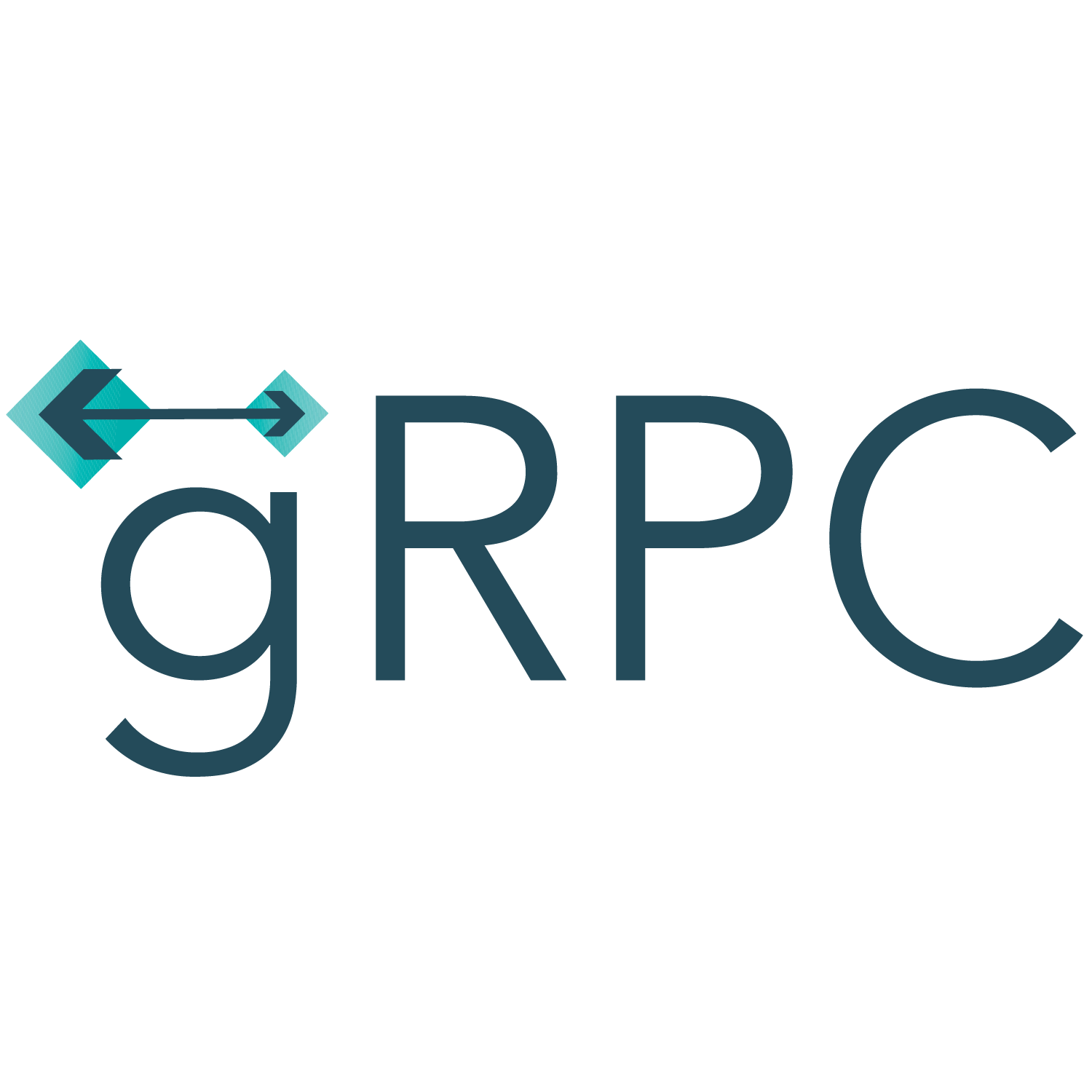
Conclusion
In conclusion, gRPC is a powerful and flexible RPC framework that offers high performance, strong typing, interoperability, and security. It is an ideal choice for building modern, cloud-native applications and microservices that require efficient and scalable communication between services. With its support for multiple programming languages, bi-directional streaming, and binary serialization, gRPC can significantly reduce latency and improve throughput, while providing a strongly-typed API that is easy to maintain and evolve over time. Overall, gRPC is a great choice for building distributed systems that require high performance, scalability, and interoperability.
REFERENCES AND FURTHER READING
- gRPC io
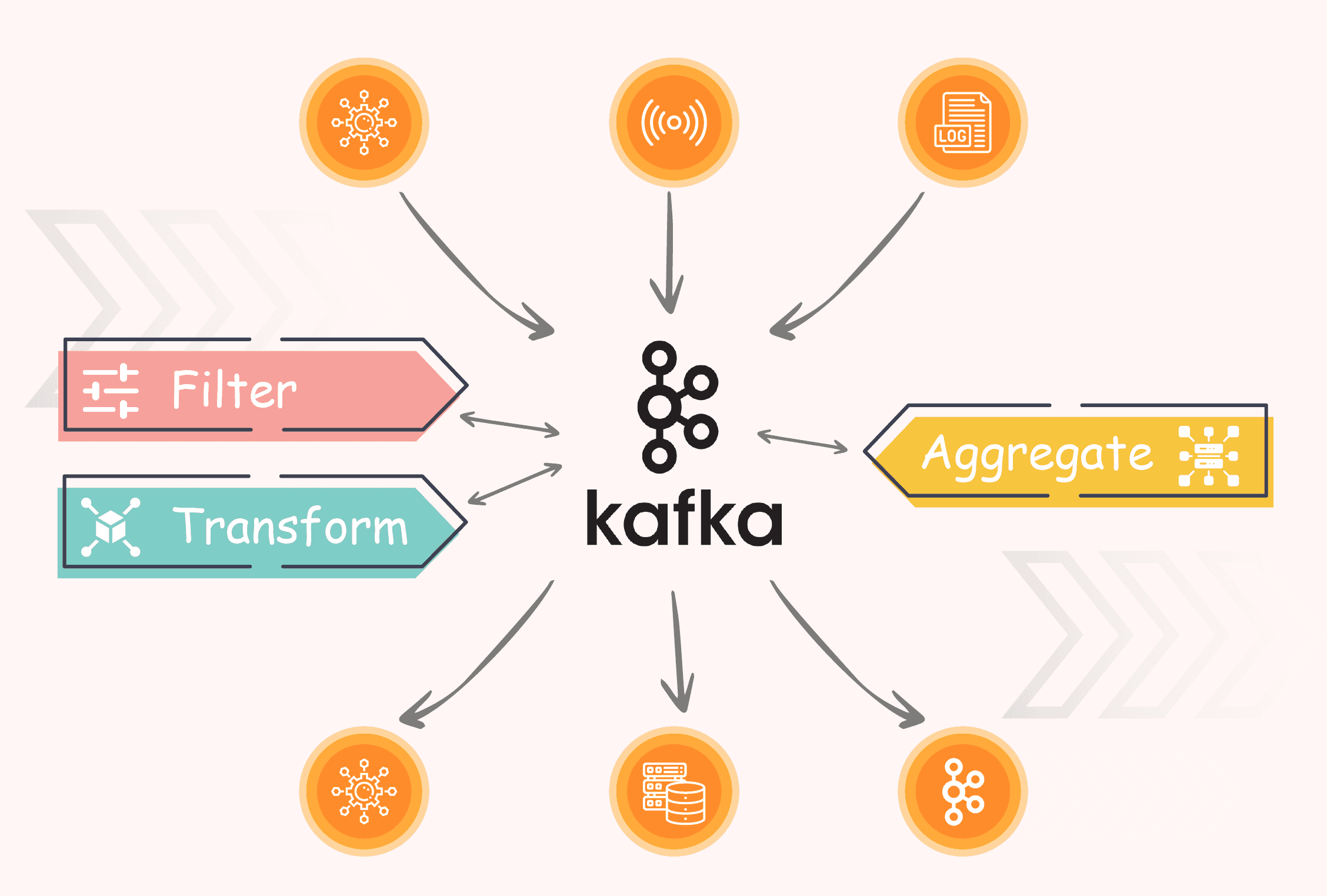